What Are They
An API, or application programming interface, is just a structured way for two applications to talk to each other. For example, your quoting engine might ask your rating engine for premiums on a quote. All APIs have this same structure: a client-side application sending a request, and a server-side application sending a response. The content of an API request or response is called its payload.
Documentation
APIs should have documentation: thorough, accurate, up-to-date docs in plain English, with working examples, aimed at someone new to the API, for everything the API does. This might sound like a trivial implementation detail, but coding with shoddy documentation is like running through knee-deep seawater: slow and difficult. At best your team will have to find the right people to talk to about this or that critical functionality. At worst, they won't find what they need at all, even though the functionality may be there. Or you'll be indefinitely dependent on integration specialists because they alone know the tool.
The need for documentation goes for anything you build on your own as well. Think of your team today as a vendor, and your team tomorrow as their customer. Have them write documentation such that tomorrow's team thanks them—even if tomorrow's team is themselves!
We've seen three kinds of good documentation in the policy software vendor space.
- Some vendors we've spoken with document their APIs publicly: You can simply head to their website and read it today.
- Others have documentation, but ask you to contact the vendor first.
- In some cases (e.g. PolicyFly) this is because their internal data structure is proprietary.
- In others they may simply want to gauge interest. If you're far enough along to be looking at documentation, it's natural to want to talk to you.
- Still others offer dynamic documentation. The API takes shape as you build your insurance product. So the structure's different for each insurer, but documentation is generated on the fly to match it.
Vendor | Type of Docs | Docs | Info |
---|---|---|---|
Boost | Public | Docs | - |
Dais | Public | Docs | - |
Insly | Dynamic | - | Info |
INSTANDA | Dynamic | - | - |
InsureMO | Public | Docs | Info |
Joshu | Dynamic | - | - |
PolicyFly | Contact Vendor | - | - |
Socotra | Public | Docs | - |
Solartis | Contact Vendor | - | Info |
In the case of InsureMO, you simply have to sign up for a free account on their portal.
All vendors we've included so far said their APIs are fully documented. We look forward to rounding out this good news with first-hand reports from customers.
Protocols
APIs in general follow one of several protocols that have developed over the years. For example:
- SOAP/XML
- JSON/REST
- GraphQL
- gRPC
- tRPC
This is by no means an exhaustive list of ways that clients and servers communicate, but these five are good ones to know.
SOAP/XML
Extensible Markup Language (XML) is a plain-text data structure that looks like HTML on steroids. SOAP is a communication protocol for sending and receiving XML messages. The API world (and specifically the World Wide Web Consortium) rallied around SOAP in the early 2000s, after Microsoft introduced it in the late 90s.
XML is still quite common in the data world. It especially has traction in the insurance world because of at least three influences:
- Major policy software vendors like Duck Creek and Guidewire use it.
- Insurtechs often have to integrate with traditional insurers, who may have their interfaces written for XML.
- Many vendors and insurers support the ACORD XML format. Among other things, the Association for Cooperative Operations Research and Development (ACORD) works to standardize insurance data interfaces so the industry isn't splintered into a thousand disparate formats.
JSON/REST
JavaScript Object Notation (JSON) is a minimal, elegant data format used by much of the internet today. You can spend just a few minutes on the json.org website and learn everything there is to know about the specs. It's native to JavaScript, as the name suggests, but it also translates directly to dictionaries and lists in another common language, Python. Because JSON is so simple and ubiquitous, most any language your team uses will have lightweight libraries to work with it. Bottom line: XML is a perfectly valid, robust format, but your team may prefer to work with JSON.
Representational State Transfer, or REST, is the architectural style most web APIs are built on today. In general, not all REST APIs are JSON—you could just as easily have an XML payload—but all JSON APIs use REST. REST protocols are generally considered simpler to implement and easier to debug than SOAP.
gRPC
gRPC is an API structure open-sourced by Google in 2015 and used by teams at Netflix, Cisco, and Square. It supports 11 common languages, it's very fast, and it allows for bi-directional data transfer.
Protocols We Haven't Seen from Vendors
There are a few other formats we have our eye on but haven't seen get any adoption in the policy software space. We mention them in case you'd like to use them in your own builds.
GraphQL
GraphQL is a more recent API protocol, developed by Facebook in 2012 and released publicly in 2015. It offers a concise, highly flexible way to query data from a server. The name is inspired by SQL, or Structured Query Language, used to get data from databases since the 70s.
tRPC
tRPC is a lightweight, performant way to structure APIs in JavaScript. The tRPC product team puts a lot of thought into providing a delightful developer experience and making it easy for teams to iterate rapidly. Still, tRPC is the only of these 5 protocols to be language-dependent. So while tRPC has been gathering momentum in JavaScript and Typescript developer communities, we have yet to see it embraced externally by a policy software vendor.
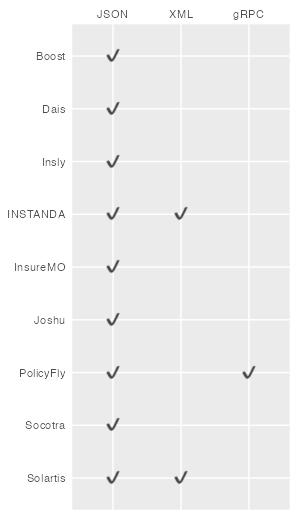
APIs and Data
Beyond production, it's common to save your API payloads for later data storage and analysis. For example, you'll likely want to store all the information you used to underwrite and rate each risk, along with your underwriting decision and premiums. One of the easiest ways to capture all that information is to hold onto your rating engine's request and response payloads.
This means that the data structures you use for your API payloads—JSON, XML, or something else—are the same structures your data and analytics team will be mining to inform and improve your business. Big-name providers like Snowflake and Databricks have functionality to read both JSON and XML data. But if you have a data team, we highly recommend involving them in any conversations you have around API design and selection.